|
orca-robotics
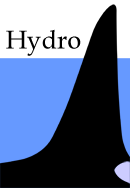
INTRODUCTION
Overview
Download and Install
Documentation
REPOSITORY
Interfaces
Drivers
Libraries
Utilities
Software Map
DEVELOPER
Dashboard
PEOPLE
Contributors
Users

Project
Download
Mailing lists
|
|
|
hydroutil Namespace ReferencelibHydroUtil provides classes for defining Hydro driver and algorithm interfaces.
More...
|
Classes |
class | Context |
| Driver's properties, tracer, status etc. More...
|
class | CpuStopwatch |
| times user-space CPU time More...
|
class | History |
| Experimental! Storage of historical information about component's activities. More...
|
class | Properties |
| Container for key-value pairs, useful for storing configuration properties. More...
|
class | RealTimeStopwatch |
| times real time More...
|
class | Stopwatch |
| base class for timing something. More...
|
class | Uncopyable |
| Handy way to avoid unintended copies. More...
|
String Utilities |
std::vector< std::string > | toStringSeq (const std::string &s, const char delim=':') |
| Parses the string into a sequence of strings with a given separator.
|
std::string | toString (const std::vector< std::string > &seq, const char delim=':') |
| Combines the sequence of strings into a single string using a given separator.
|
std::string | toLowerCase (const std::string &s) |
| Converts the whole string to lower case.
|
std::string | toUpperCase (const std::string &s) |
| Converts the whole string to upper case.
|
int | toIntVector (const std::string &s, std::vector< int > &obj) |
int | toDoubleVector (const std::string &s, std::vector< double > &obj) |
std::string | toFixedWidth (const std::string &s, int width, char filler, bool adjustLeft) |
std::string | orcaVersion () |
| Returns version of libOrcaIce, e.g. "3.2.1".
|
std::string | basename (const std::string &path, bool removeExtension) |
std::string | dirname (const std::string &path) |
void | substitute (std::string &s, const std::vector< std::string > ¶meters, const std::map< std::string, std::string > &values, const std::map< std::string, std::string > &defaults) |
System-Dependent Functions |
string | getHostname () |
string | pathDelimeter () |
| Returns "/" in Linux, "\" in Windows.
|
bool | executeSystemCommand (const std::string &command, std::string &failReason, std::string *output=NULL) |
Functions |
bool | outputMatchesReferenceFile (const std::string &outputString, const std::string &referenceFileName) |
double | normalRand (double mean, double std) |
| Normally-distributed random number.
|
double | randNum (double minVal, double maxVal) |
| returns a random number in the range [minVal,maxVal)
|
double | randNumInclusive (double minVal, double maxVal) |
| returns a random number in the range [minVal,maxVal]
|
double | G (double dist, double sd) |
| Returns the height of a 1D gaussian at 'dist' from the mean.
|
double | GCov (double dist, double cov) |
| Returns the height of a 1D gaussian at 'dist' from the mean.
|
double | multiG (double xDiff, double yDiff, double xx, double yy) |
std::vector< std::string > | tokenise (const std::string &str, const std::string &delimiter) |
Detailed Description
libHydroUtil provides classes for defining Hydro driver and algorithm interfaces.
This namespace is part of a library.
- See also:
- libHydroUtil
Function Documentation
std::string hydroutil::basename |
( |
const std::string & |
path, |
|
|
bool |
removeExtension = false |
|
) |
|
|
|
Similar to UNIX basename command. Removes the path, i.e. all leading characters up to the path delimeter ('/' in Linux, '\' in Windows). E.g. basename("/path/filename.ext") returns "filename.txt". Unlike the Unix equivalent, this function returns an empty string when the intput string is a directory name. E.g. basename("/path/" ) returns "". If the optional flag removeExtension is set to TRUE, then all trailing characters following and including the last '.' are removed as well. E.g. basename("/path/filename.ext", true) returns "filename". |
std::string hydroutil::dirname |
( |
const std::string & |
path |
) |
|
|
|
Similar to UNIX dirname command. Removes the trailing characters following and including the last path delimeter ('/' in Linux, '\' in Windows). E.g. dirname("/path/filename.ext") returns "/path". If the path delimeters are absent, returns ".". |
bool hydroutil::executeSystemCommand |
( |
const std::string & |
command, |
|
|
std::string & |
failReason, |
|
|
std::string * |
output = NULL |
|
) |
|
|
|
Returns:
- True: success
- False: failure (and failReason is set)
On Linux calls popen : opens a process by creating a pipe, forking, and invoking the shell (see: man popen). Grabs both stdout and stderr. |
std::string hydroutil::getHostname |
( |
|
) |
|
|
|
Platform-independent function to get the hostname. On error, returns localhost.
- Note:
- only Linux version is implemented, under Windows returns localhost.
|
double hydroutil::multiG |
( |
double |
xDiff, |
|
|
double |
yDiff, |
|
|
double |
xx, |
|
|
double |
yy |
|
) |
[inline] |
|
|
The value of the two-dimensional axis-aligned gaussian with covariance xx,xy,yy, at the point 'diffs'. |
bool hydroutil::outputMatchesReferenceFile |
( |
const std::string & |
outputString, |
|
|
const std::string & |
referenceFileName |
|
) |
|
|
|
Writes the outputString to a file named "test_output.txt", then compares the contents of that file to the contents of referenceFileName. Returns true if it matches. |
void hydroutil::substitute |
( |
std::string & |
s, |
|
|
const std::vector< std::string > & |
parameters, |
|
|
const std::map< std::string, std::string > & |
values, |
|
|
const std::map< std::string, std::string > & |
defaults |
|
) |
|
|
|
Performs parameter substitution in string the string provided. Parameter syntax: ${parameter_name}. Valid parameters are given in parameters . Substitution values are first looked up in values and then in defaults . Throws gbxsickacfr::gbxutilacfr::Exception if the string conatains an unknown parameter or if the parameter has no value (provided or default).
Substitution can be recursive, provided that enough information is provided and the dependencies are not circular. For example var1.value="a" , var2.default=$var1" . Be careful, circular dependencies are not detected. For example, this will lead to an infinite loop: var1.value="$var2" , var2.value=$var1" .
The input string can contain '${' without a matching '}'. These are ignored.
Example: string s="The best framework is ${framework}";
vector<string> parameters;
parameters.push_back("software");
parameters.push_back("framework");
map<string,string> values;
map<string,string> defaults;
defaults["framework"] = "Orca";
substitute( s, parameters, values, defaults );
cout<<s<endl; // output: "The best framework is Orca"
|
int hydroutil::toDoubleVector |
( |
const std::string & |
, |
|
|
std::vector< double > & |
|
|
) |
|
|
|
Parses string of doubles separated by spaces. Returns: 0 = parsing successful, non-zero = parsing failed. |
std::string hydroutil::toFixedWidth |
( |
const std::string & |
s, |
|
|
int |
width, |
|
|
char |
filler = ' ' , |
|
|
bool |
adjustLeft = false |
|
) |
|
|
|
Unlike std::setw() function, this functions pads and truncates. When width=0, an empty string is returned. When width<0, the string is quietly returned unmodified. Default fill character is a space. |
int hydroutil::toIntVector |
( |
const std::string & |
, |
|
|
std::vector< int > & |
|
|
) |
|
|
|
Parses string of int's separated by spaces. Returns: 0 = parsing successful, non-zero = parsing failed. |
std::vector<std::string> hydroutil::tokenise |
( |
const std::string & |
str, |
|
|
const std::string & |
delimiter |
|
) |
|
|
|
Takes a string containing tokens separated by a delimiter Returns the vector of tokens |
|
|